Have you ever wanted to add some fun animations and graphics into a React Native app? Lottie is a a native library for adding Adobe After Effects animations into your app.
Of course, there's already an open source library for adding Lottie animations to React Native apps - lottie-react-native.
In this video, I'll give a quick walk through of how to add some fun animations to your app.
Here's a link to try it out in your own Expo app: https://expo.io/@calendee/expo-template-bare and a QR code to scan:
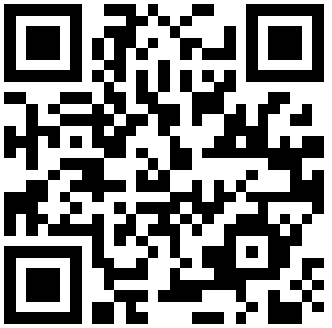
Here are the official Expo docs for Lottie. This is where I got much of the code for this demo.
Any my sample code:
import React, { useEffect, useRef, useState} from 'react';
import { Button, StyleSheet, View } from 'react-native';
import LottieView from "lottie-react-native";
import RedChristmasTree from './assets/12661-red-christmas-tree.json';
import HappyChristmasTree from './assets/12682-happy-christmas';
import SantaClaus from './assets/12720-santa-claus-loading-animation.json';
import MerryChristmas from './assets/12764-merry-christmas-text.json';
import HappyBirthday from './assets/427-happy-birthday.json';
import MerryChristmas2 from './assets/1145-merrychristmas.json';
import ChristmasTree2 from './assets/3477-christmas-tree-with-gift-boxes.json';
import Angel from './assets/3645-angel.json';
import BeforeChristmas from './assets/3992-week-before-christmas.json';
import ChrismasBounce from './assets/11517-christmas-bounce.json';
import StopHere from './assets/3711-santa-stop-here.json';
const animations = [
RedChristmasTree, HappyChristmasTree, SantaClaus,
MerryChristmas, HappyBirthday, MerryChristmas2,
ChristmasTree2, Angel, BeforeChristmas, ChrismasBounce, StopHere
];
const App = () => {
const [currentAnimation, setCurrentAnimation] = useState();
const animation = useRef(null);
useEffect(() => {
if (animation.current) {
animation.current.play();
}
}, [currentAnimation]);
const changeAnimation = () => {
const newAnimation = Math.floor(Math.random() * animations.length);
setCurrentAnimation(animations[newAnimation]);
}
if (!currentAnimation) {
return (
<View style={styles.animationContainer}>
<Button title="Start!" onPress={changeAnimation} />
</View>
)
}
return (
<View style={styles.animationContainer}>
<LottieView
ref={animation}
style={{
width: 400,
height: 400,
backgroundColor: '#eee',
}}
source={currentAnimation}
/>
<Button title="Change Animation" onPress={changeAnimation} />
</View>
)
}
export default App;
const styles = StyleSheet.create({
animationContainer: {
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
flex: 1,
},
buttonContainer: {
paddingTop: 20,
},
});