Today, I was spinning up a new Expo React Native project and realized I didn't have my favorite tool installed on my personal machine - Reactotron.
I've used Reactotron for several years on work projects, but just never got around to installing it on my side projects. I assumed that getting it to work in an Expo project would be difficult. How wrong I was! Here is all you need to do:
Installation and Setup
Step 1: Add a ReactotronConfig.js
file to the project:
import Reactotron from "reactotron-react-native";
import { AsyncStorage } from "react-native";
Reactotron.setAsyncStorageHandler(AsyncStorage)
.configure({
name: "Reactotron In Expo demo",
})
.useReactNative({
asyncStorage: false,
networking: {
ignoreUrls: /symbolicate|127.0.0.1/,
},
editor: false,
errors: { veto: stackFrame => false },
overlay: false,
})
.connect();
NOTE: The line ignoreUrls: /symbolicate|127.0.0.1/,
really helps keep Reactotron timeline quieter. Otherwise, all the traffic between the app and Expo that logs to the terminal will also log to Reactotron.
Step 2: Import the Reactron configuration into App.tsx
:
if (__DEV__) {
import("./ReactotronConfig").then(() => console.log("Reactotron Configured"));
}
Step 3: Add some sample Reactotron logging into App.tsx
:
import Reactotron from "reactotron-react-native";
...
...
<Button
title="Test Log"
onPress={() => {
Reactotron.log("hello rendering world");
}}
></Button>
<Button
title="Test Warning"
onPress={() => {
Reactotron.warn("I'm warning you!");
}}
></Button>
<Button
title="Test Error"
onPress={() => {
Reactotron.error("Now you're in trouble!", null);
}}
></Button>
<Button
title="Test Display"
onPress={() => {
Reactotron.display({
name: "KNOCK KNOCK",
preview: "Who's there?",
value: "Orange.",
});
Reactotron.display({
name: "ORANGE",
preview: "Who?",
value: "Orange you glad you don't know me in real life?",
important: true,
});
}}
></Button>
Step 4: Watch your Reactotron timeline as you tap the new buttons:
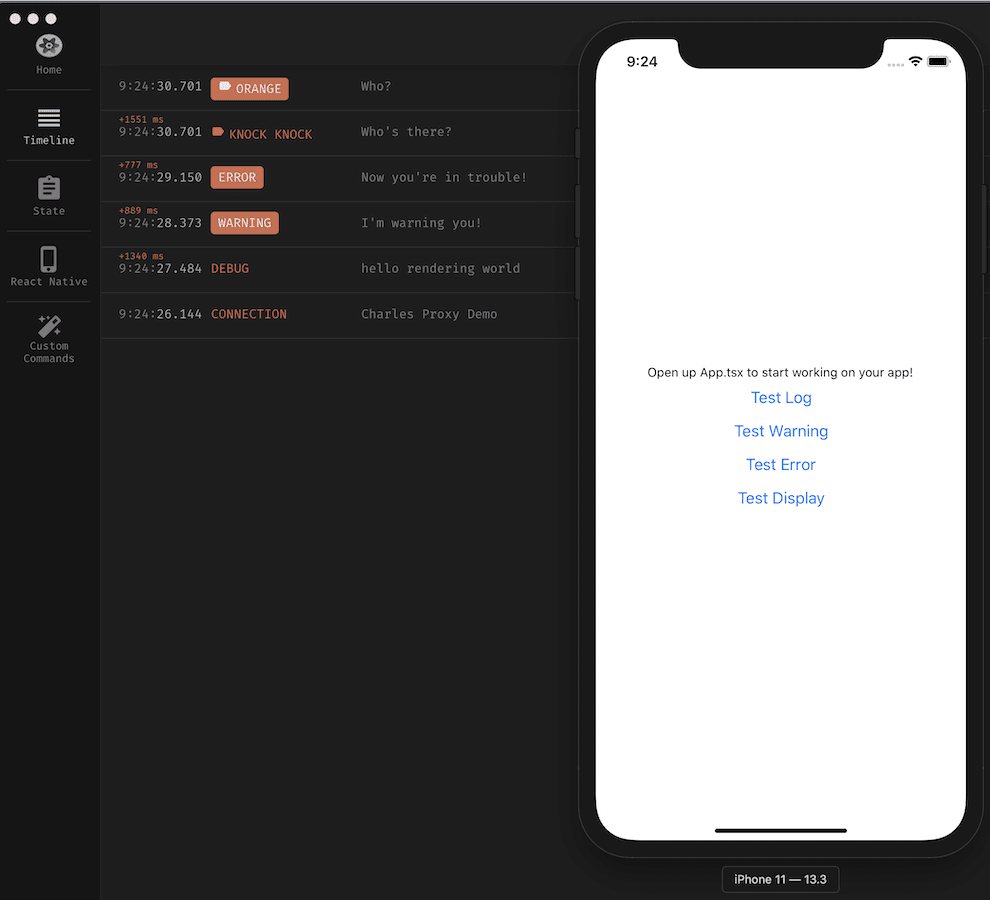