While working on Kids In Touch today, I needed to implement "pull to refresh" on a user's friends list. I had been putting it off because I thought it'd be a bit of a nightmare.
After reviewing the Ionic Framework documentation, I saw this was going to be very simple. In about 10 minutes, I had pull to refresh working on multiple pages.
Here's a sample :
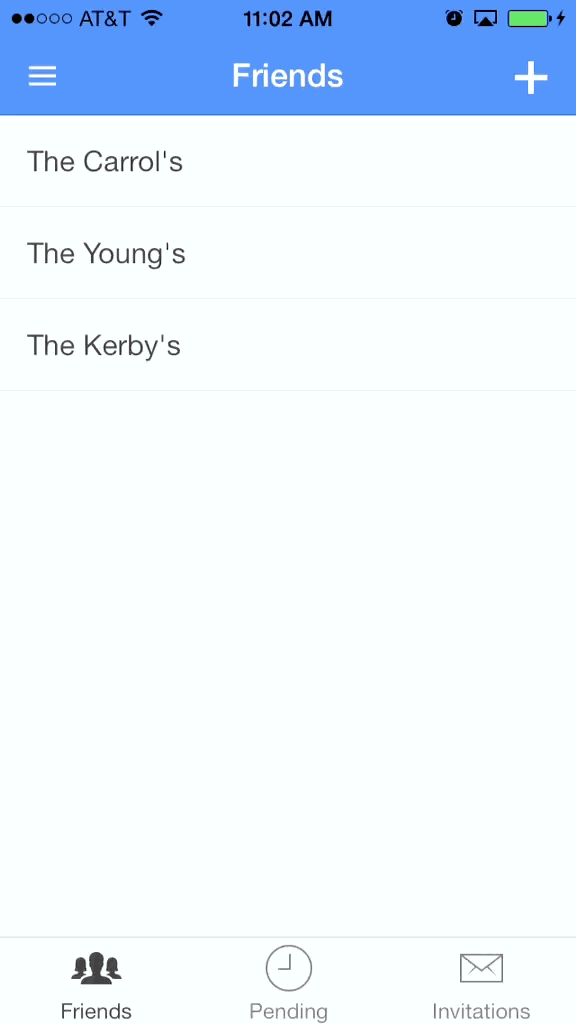
The code to accomplish PTR is really simple:
<tab title="Friends" icon-on="icon ion-ios7-people" icon-off="icon ion-ios7-people-outline">
<content has-header="true" on-refresh="refreshFriends()">
<refresher></refresher>
<div ng-if="fc.friends.length === 0" class="padding">
<h1>Got Friends?</h1>
<p>Looks like you could use some company here.</p>
<p>Tap the plus sign to invite some other families.</p>
</div>
<div class="list" ng-if="fc.friends.length > 0">
<div class="item" href="#" ng-repeat="friend in fc.friends">
The {{friend.familyName}}'s
</div>
</div>
</content>
</tab>
$scope.refreshFriends = function() {
FriendsService.flushFriendsCache();
FriendsService.getFriends().then(
function(friendDetails) {
$scope.$broadcast('scroll.refreshComplete');
$scope.fc.friends = friendDetails;
},
function(failure) {
$scope.$broadcast('scroll.refreshComplete');
}
);
};